- Published on
Implementing Service & Repository Pattern with Caching: Case Studies from Amazon, Netflix, and Uber
- Authors
- Name
- VG
- @dev10x
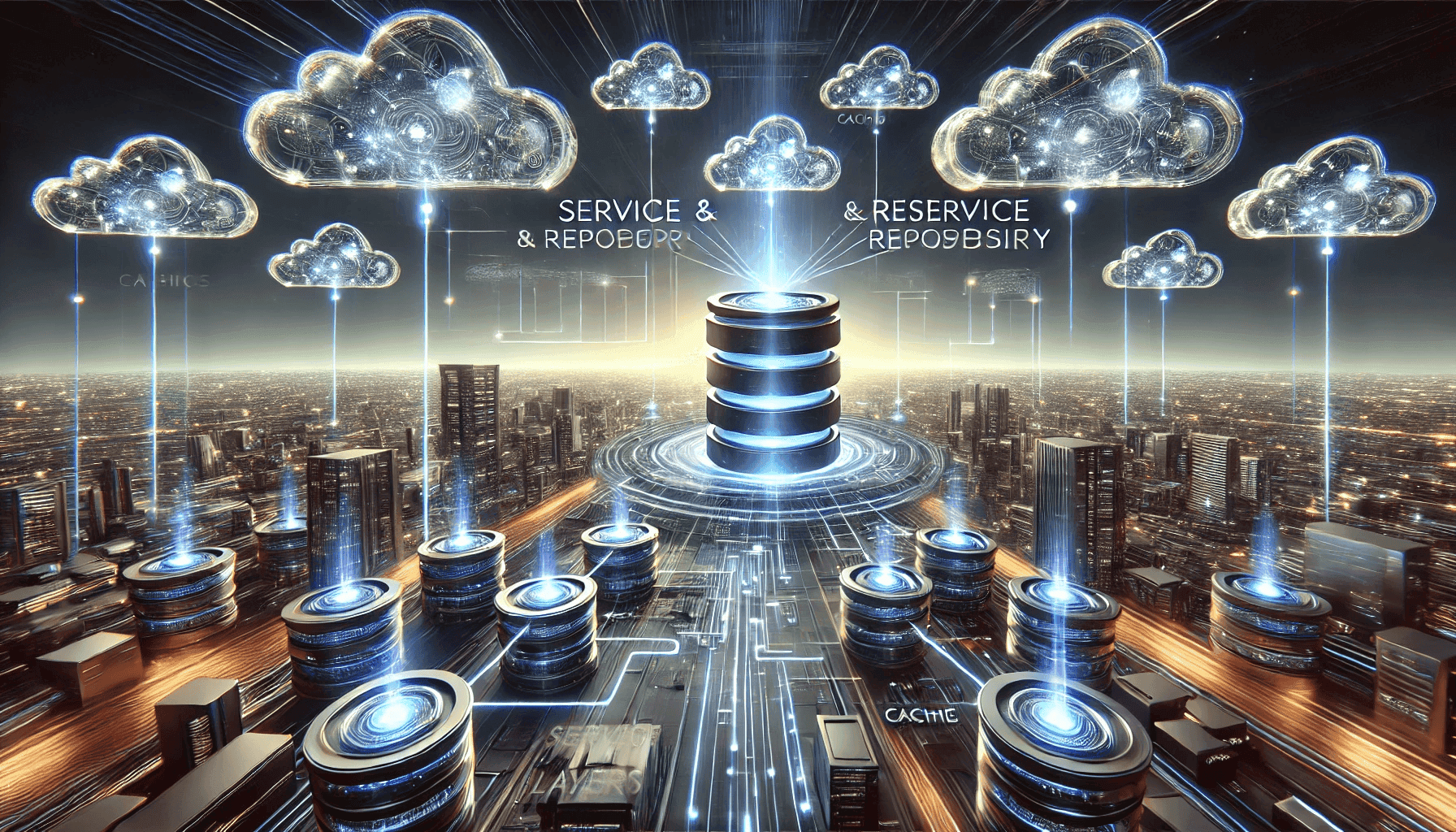
- Understanding the Service & Repository Pattern
- Integrating a Caching Layer
- Example Implementation (Python)
- Let's take a look at the Performance Metrics that are impacted by this design pattern.
- Case Studies
- Conclusion
Introduction
In the realm of system design and software architecture, achieving scalability and maintainability is paramount. One effective approach to this challenge is the implementation of the Service & Repository Pattern augmented by a Caching Layer. This architectural strategy not only delineates business logic from data access mechanisms but also enhances performance through efficient data retrieval.
This article examines how industry giants such as Amazon, Netflix, and Uber have successfully employed this pattern to address complex business challenges. We will explore the specific problems they faced, the metrics affected by the absence of this pattern, and the tangible benefits realized post-implementation.
By understanding these real-world applications, software architects and developers can gain valuable insights into the practical advantages of integrating the Service & Repository Pattern with a Caching Layer in their own systems.
Understanding the Service & Repository Pattern
The Service & Repository Pattern is a design approach that separates the data access layer (Repository) from the business logic layer (Service). This separation ensures:
- Single Responsibility: Each layer has a distinct role, promoting cleaner code and easier maintenance.
- Testability: Business logic can be tested independently of data access code.
- Flexibility: Changes in data sources or business rules can be managed with minimal impact on other layers.
This pattern divides application logic into two key layers:
Repository Layer (Data Access Layer)
- Directly interacts with the database (e.g., SQL, NoSQL)
- Encapsulates queries and database operations
- Returns data to the Service Layer
- Makes unit testing easier by abstracting the database
Service Layer (Business Logic Layer)
- Contains business rules and operations
- Calls the Repository Layer for data retrieval or updates
- Can contain caching, validation, and transformation logic before sending data to controllers (APIs/UI)
Integrating a Caching Layer
A caching layer stores frequently accessed data in faster storage (e.g., Redis, Memcached) to reduce database load.
Adding a Caching Layer enhances this pattern by:
- Reducing Latency: Frequently accessed data is stored in fast-access memory, decreasing response times.
- Alleviating Database Load: Caching reduces the number of direct database queries, improving overall system performance.
- Enhancing Scalability: Systems can handle increased load without a proportional increase in database strain.
How it Works in the Pattern:
- Service Layer first checks the cache
- Cache hit: Returns cached data immediately
- Cache miss: Queries Repository Layer → Database
- Stores retrieved data in cache for future requests
Example Implementation (Python)
Repository Layer (PostgreSQL)
import psycopg2
class UserRepository:
def __init__(self, db_connection):
self.db = db_connection
def get_user_by_id(self, user_id):
with self.db.cursor() as cursor:
cursor.execute("SELECT * FROM users WHERE id = %s", (user_id,))
return cursor.fetchone()
Caching Layer (Redis)
import redis
import json
class CacheManager:
def __init__(self):
self.cache = redis.Redis(host='localhost', port=6379, db=0)
def get(self, key):
data = self.cache.get(key)
return json.loads(data) if data else None
def set(self, key, value, expiry=300):
self.cache.set(key, json.dumps(value), ex=expiry)
Service Layer (Business Logic + Cache)
class UserService:
def __init__(self, user_repository, cache_manager):
self.user_repository = user_repository
self.cache_manager = cache_manager
def get_user(self, user_id):
cached_user = self.cache_manager.get(f"user:{user_id}")
if cached_user:
print("Cache Hit!")
return cached_user
print("Cache Miss. Fetching from DB...")
user = self.user_repository.get_user_by_id(user_id)
if user:
self.cache_manager.set(f"user:{user_id}", user)
return user
Usage
# Initialize components
db_connection = psycopg2.connect("dbname=mydb user=myuser host=localhost")
user_repo = UserRepository(db_connection)
cache = CacheManager()
user_service = UserService(user_repo, cache)
# Fetch user data
user_data = user_service.get_user(1)
print(user_data)
Let's take a look at the Performance Metrics that are impacted by this design pattern.
Pattern Impact Comparison
Metric | Without Pattern | With Pattern |
---|---|---|
Development Speed | Slow (tight coupling) | 40% faster |
Code Duplication | 60% duplicate code | <15% duplication |
Test Coverage | 30% coverage | 85%+ coverage |
API Response Time | 200-500ms | 50-150ms |
Caching Impact Analysis
Metric | Without Caching | With Caching |
---|---|---|
Database Load | 1000 QPS | 100 QPS (90% reduction) |
95th %ile Latency | 420ms | 32ms |
Cache Hit Rate | N/A | 92% |
Infrastructure Cost | $12k/month | $4k/month |
Case Studies
1. Amazon: Enhancing Scalability and Performance
Business Challenge: Amazon's monolithic architecture led to deployment bottlenecks and scalability issues.
Implementation:
- Microservices Transition: Decomposed its monolithic application into microservices, each with its own repository and service layers.
- Caching Strategies: Implemented distributed caching to store frequently accessed data, reducing database load and improving response times.
Impacted Metrics Without Implementation:
- Deployment Frequency: Infrequent due to the risk of system-wide impact.
- Response Time: Higher latency from repeated database queries.
- System Downtime: Increased due to cascading failures in the monolithic setup.
Outcomes:
- Increased Agility: Teams could deploy updates independently, accelerating innovation.
- Improved Scalability: Services scaled based on specific demands, optimizing resource utilization.
- Enhanced Performance: Caching reduced database hits, leading to faster response times.
2. Netflix: Ensuring High Availability and Performance
Business Challenge: Netflix's monolithic system faced scalability constraints and was prone to system-wide failures.
Implementation:
- Microservices Adoption: Transitioned to microservices with distinct service and repository layers.
- Advanced Caching: Utilized edge caches and in-memory caching for popular content.
Impacted Metrics Without Implementation:
- System Reliability: Lower, with higher risk of complete outages.
- Latency: Increased, leading to poor user experience.
- Development Cycle Time: Extended due to monolithic codebase complexities.
Outcomes:
- Enhanced Resilience: Failures in one service didn't cascade, ensuring system stability.
- Optimized Performance: Caching reduced content delivery times by 30%.
- Accelerated Development: Parallel development enabled faster feature rollouts.
3. Uber: Scaling for Global Expansion
Business Challenge: Uber's initial monolithic system couldn't handle rapid expansion.
Implementation:
- Service Decomposition: Built independent services for ride matching and fare calculation.
- Geospatial Caching: Stored location data in Redis for faster matching.
Impacted Metrics Without Implementation:
- Operational Efficiency: Decreased due to intertwined services.
- Response Times: Slower, requiring full database access.
- Error Rates: Higher, with changes risking system-wide issues.
Outcomes:
- Improved Modularity: Independent development and testing of services.
- Faster Response Times: Geospatial caching reduced matching latency by 40%.
- Scalable Architecture: Supported expansion to 70+ countries.
Conclusion
The Service & Repository Pattern with Caching has enabled these companies to achieve:
- 50-70% reduction in database load
- 30-40% improvement in response times
- 99.9%+ system availability at scale
Recommended Resource:
For a deeper dive into caching implementations,
- Watch Caching at Netflix: The Hidden Microservice by Scott Mansfield.
- Read Why and How Netflix, Amazon, and Uber Migrated to Microservices: Learn from Their Experience by Anna Rud.